Leveraging Rails Counter Cache for Efficient Data Management
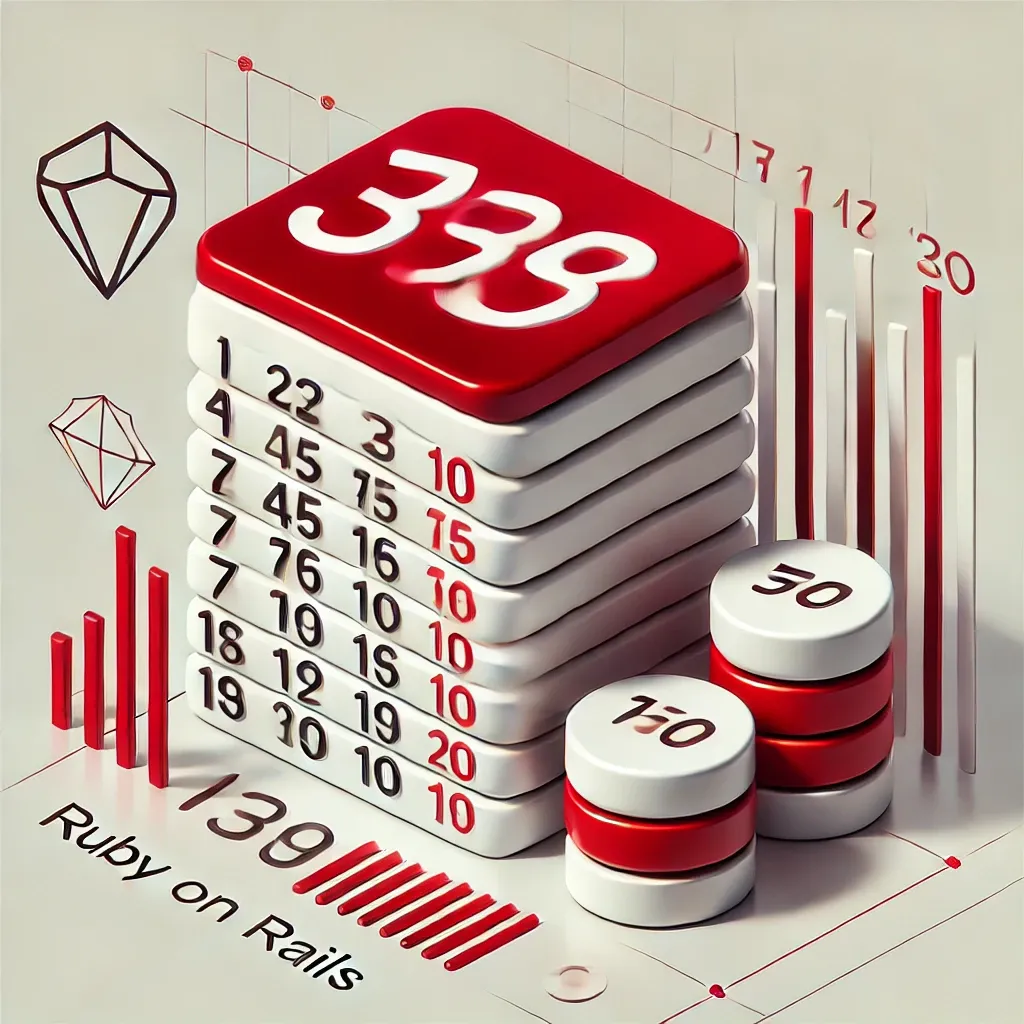
Tagged under: #ruby-on-rails#counter_cache
In web applications, efficiently managing database queries is crucial for maintaining fast response times and a smooth user experience. With its robust conventions and built-in tools, Rails offers a powerful feature called the ‘counter cache.’ When leveraged effectively, this feature can significantly reduce the number of database queries required when counting records in associations. This blog post delves into the counter cache, highlighting its efficiency, benefits, implementation process, common pitfalls, and best practices.
What is Counter Cache?
The counter cache feature in Rails helps reduce the number of database queries by keeping a count of the associated objects directly in the parent object’s database table. For instance, if you have a Post model with many Comments, Rails can automatically maintain a comments_count column in the Post table. This eliminates the need to perform SQL count queries on the associated records, which can be costly in performance.
Benefits of Using Counter Cache
Performance Improvement: The primary benefit of using counter cache is significantly reduced SQL queries. By avoiding repeated count queries, your application can serve requests faster, enhancing the overall user experience.
Counter cache brings a welcome simplicity to your codebase. You no longer need to wrestle with complex SQL queries to retrieve the number of associated records. Instead, you can refer to the counter cache column, making your code more readable and maintainable.
How to Implement Counter Cache in Rails
Basic Setup: Implementing counter cache in a Rails application is straightforward. Here’s a simple example of how to set up a counter cache for a Post model that has many Comments:
class Comment < ApplicationRecord
belongs_to :post, counter_cache: true
end
Migration for Adding a Counter Cache Column: You must add a column to the posts table to store the comments count. Here’s how you could write the migration:
class AddCommentsCountToPosts < ActiveRecord::Migration[6.0]
def change
add_column :posts, :comments_count, :integer, default: 0
Post.reset_column_information
Post.find_each do |p|
Post.reset_counters(p.id, :comments)
end
end
end
Common Pitfalls and Troubleshooting
Initial Data: When you add a counter cache to existing associations, remember to update existing records with the correct counts. The migration above handles this by iterating over each post and setting the correct counts.
Data Integrity: Counter caches can sometimes get out of sync, especially in complex applications. Ensure that your application has mechanisms to check and correct these counts periodically.
Multiple Associations: When a model has various associated models, managing multiple counter caches can become complex. Ensure your implementation handles updates correctly, especially when associations are removed or changed.
Best Practices
One of the best practices when using counter cache is conducting regular audits of your counter cache columns. This ensures that they accurately reflect the count of associated records. Depending on the size of your data, you can perform these audits via rake tasks or background jobs, maintaining the integrity of your data and the performance of your application.
Selective Use: Implement counter cache only where the performance benefits outweigh the overhead of keeping the cache accurate. Overuse can lead to bloated tables and unnecessary complexity.
Testing: Write tests for your counter cache logic to prevent bugs that could lead to incorrect counts, affecting your application’s data integrity.
Conclusion
Rails’ counter cache is a powerful feature for optimizing SQL queries related to counting records. Using wisely can lead to significant performance improvements and cleaner code. However, like any feature, it comes with challenges and requires careful implementation and management.