Demystifying Ruby on Rails Routes for Beginners
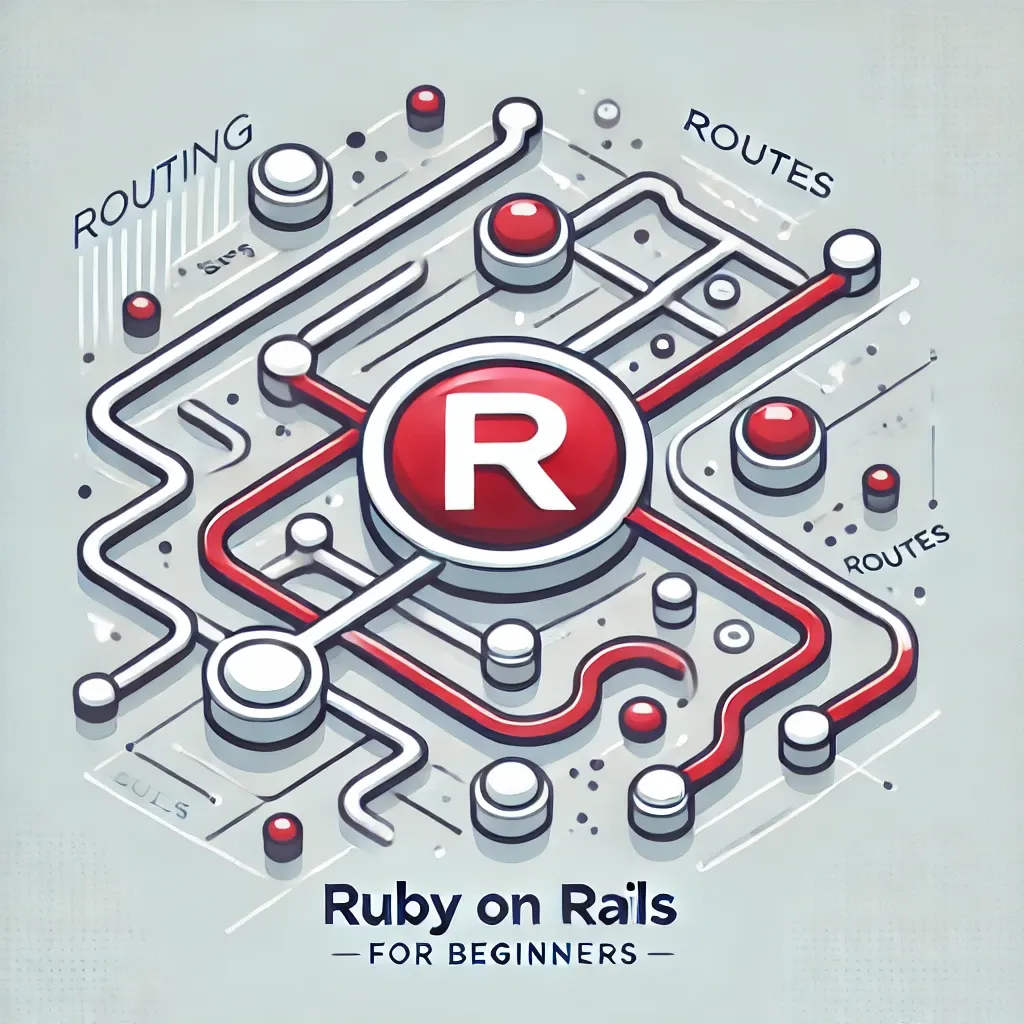
Tagged under: #ruby-on-rails#ruby
Routes in Rails determine how URLs are mapped to specific controller actions. When a user sends a request to your application, the routing system will parse the URL and match it to a controller action. This action is responsible for processing the request and returning an appropriate response.
Routes are defined in the config/routes.rb
file within your Rails application.
Let’s look at a simple example:
Rails.application.routes.draw do
get 'welcome/index', to: 'welcome#index'
end
In this example, we’ve created a route that maps a GET request to the welcome/index
URL to the index
action of the WelcomeController
.
Resource vs Resources
Now that we understand the basics of routing, let’s discuss the difference between resource
and resources
in Rails.
Resource
The resource
method creates routes for a singular resource, such as a user profile or a shopping cart. This means that it will only generate routes that do not require an ID to be passed in the URL. The generated routes will include new, create, show, edit, update, and destroy actions.
Here’s an example:
Rails.application.routes.draw do
resource :profile
end
This will generate the following routes:
new_profile GET /profile/new profiles#new
edit_profile GET /profile/edit profiles#edit
profile GET /profile profiles#show
PATCH /profile profiles#update
PUT /profile profiles#update
DELETE /profile profiles#destroy
POST /profile profiles#create
Resources
The resources
method is used when you want to create routes for a collection of resources, such as blog posts or products. It generates routes for all seven default RESTful actions: index, new, create, show, edit, update, and destroy.
Here’s an example:
Rails.application.routes.draw do
resources :articles
end
This will generate the following routes:
articles GET /articles articles#index
new_article GET /articles/new articles#new
edit_article GET /articles/:id/edit articles#edit
article GET /articles/:id articles#show
POST /articles articles#create
PATCH /articles/:id articles#update
PUT /articles/:id articles#update
DELETE /articles/:id articles#destroy
Notice that in the resources
method, the routes include an :id
parameter, which represents the identifier of the specific resource.
Conclusion
Understanding Ruby on Rails routes is essential for any beginner developer working with Rails applications. By mastering the difference between resource
and resources
, you’ll be able to create clean, RESTful routes that efficiently map user requests to the appropriate controller actions. Keep practicing, and soon you’ll be a Rails routing pro!