The Key Differences Between let and let! in RSpec
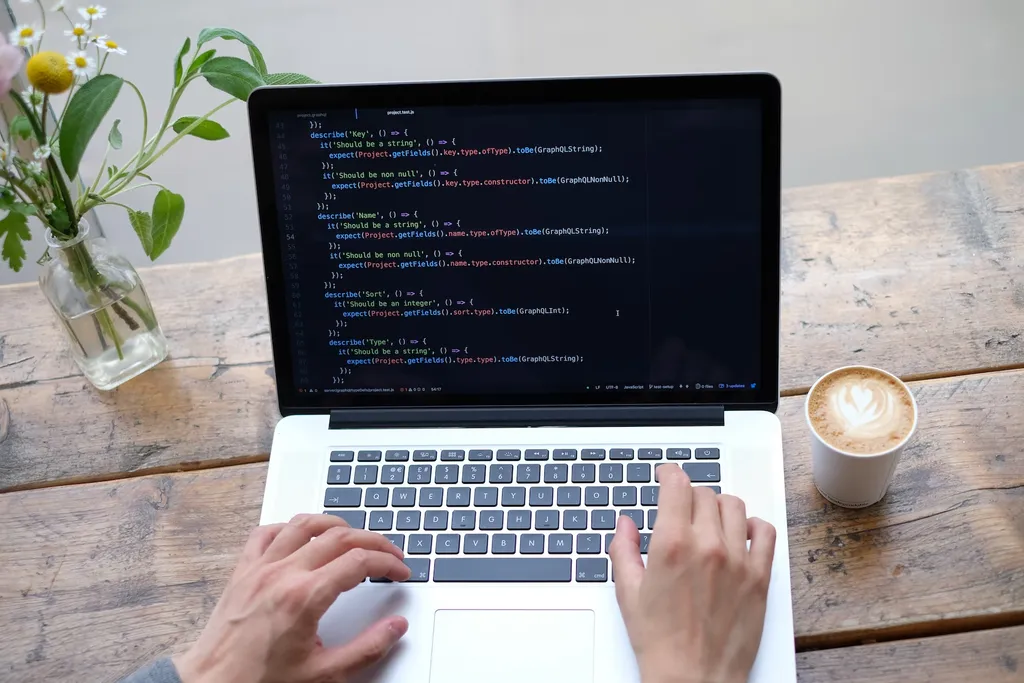
Tagged under: #rspec#ruby
In the Ruby testing framework RSpec, the let
and let!
methods are used to define a variable or method that can be used within an example or group of examples. Both let and let!
are memoized, meaning that their value will be cached and re-used within the same example or group of examples, but there are some key differences between the two that are important to understand.
One major difference between let and let!
is the order in which they are evaluated. let is lazy-evaluated, meaning that it will not be evaluated until it is called within an example. This can be useful if the value of the let variable is expensive to compute, as it will only be calculated when it is actually needed. let!
, on the other hand, is evaluated immediately, regardless of whether it is called within an example. This can be useful if the value of the let!
variable needs to be available before any examples are run.
Another difference between let and let!
is that let!
will override any previously defined let variables with the same name, while let will not. This means that if you define a let variable and then later define a let!
variable with the same name, the let!
variable will take precedence and will be used instead of the let variable.
Here is an example that demonstrates the difference between let and let!
:
describe "example group" do
let(:foo) { "foo" }
let!(:bar) { "bar" }
it "uses the value of let" do
expect(foo).to eq("foo")
end
it "uses the value of let!" do
expect(bar).to eq("bar")
end
describe "nested example group" do
let(:bar) { "baz" }
it "overrides the value of let!" do
expect(bar).to eq("baz")
end
end
end
In the above example, the foo variable is defined using let and is lazy-evaluated, while the bar variable is defined using let!
and is immediately evaluated. Within the first example, both foo and bar are accessed and their values are used as expected. However, in the nested example group, a new bar variable is defined using let, which overrides the value of the let!
variable defined in the outer example group.
In conclusion, the difference between let and let!
in RSpec is mainly in the order in which they are evaluated and the way in which they can override previously defined variables. Understanding when to use let and when to use let!
can help you write more efficient and effective tests in RSpec.